How to install Prism.js with NPM for Web
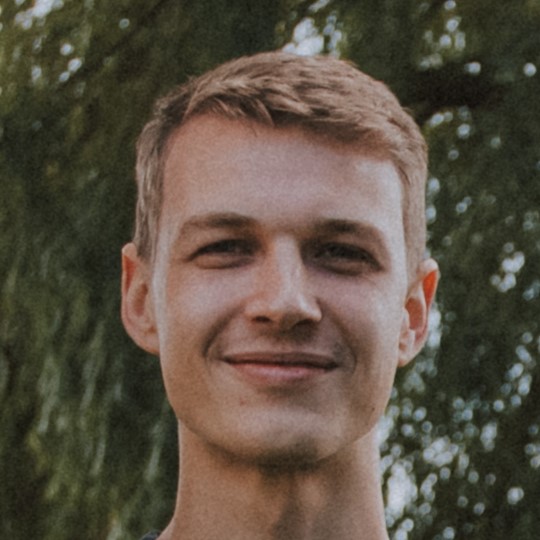
Prism.js lists various installation methods in their documentation. When I first tried installing it I was a bit confused as to how to install it using NPM, how to load themes, and how to load the languages I was trying to highlight.
This post explains how you can install Prism.js with NPM, how to load themes, and how to load languages you want to highlight.
You can use this with HTML and vanilla Javascript, Vue, React, etc.
1. Installing Prism.js
First, install Prism.js by running the following command:
npm install prismjs
Next, go to your main Javascript file and import Prism.js with the following statement:
// Import Prism.js
import 'prismjs';
2. Load the languages you want to highlight
When you import Prism.js, by default it only loads code highlighting for the following languages: "markup, css, clike and javascript".
To load different languages, use the following statement:
// Load the languages you want to highlight
// @see https://github.com/PrismJS/prism/tree/master/components for an overview of all languages
import 'prismjs/components/prism-markup-templating';
import 'prismjs/components/prism-php';
import 'prismjs/components/prism-java';
3. Load the theme (colors used for highlighting)
Next, we need to load the stylesheet used for coloring the highlighted code. There are 3 routes we can take:
3.1 Load a default theme
Prism.js ships with a few default themes, you can preview them here: (press the buttons on the right side of the screen to change the theme): https://prismjs.com/examples.html
Load the theme by using the following statement:
// Option 1: Load a default theme (included in the 'prismjs' package)
import 'prismjs/themes/prism-okaidia.css';
3.2 Load a 'prism-themes' theme
There is also a package containing a variety of extra Prism.js themes. A showcase of all of them can be found on the package's GitHub page: https://github.com/PrismJS/prism-themes#available-themes
First install the package:
npm install prism-themes
Load it with the following statement:
// Option 2: Import an extra theme (included in the 'prism-themes' package)
import 'prism-themes/themes/prism-coldark-dark.css';
3.3 Create your own theme
You can also create your own theme. You can create a CSS file manually, but there is also a generator you can use to ease the process and preview your theme:
http://k88hudson.github.io/syntax-highlighting-theme-generator/www/
After creating the theme, press the 'Download CSS' button to download your theme's CSS file, and load it:
// Option 3: Load your own theme:
import 'path/to/your/theme/your-theme-name.css';
4. Write an HTML code block
Add a class to your code
block structured as language-{language}
with block's language. This allows Prism.js to apply the language highlighting inside the block.
<pre><code class="language-javascript">const test = () => console.log('test!');</code></pre>
Results in:
const test = () => console.log('test!');
5. The complete solution
// Import Prism.js
import 'prismjs';
// Load the languages you want to highlight
// @see https://github.com/PrismJS/prism/tree/master/components for an overview of all languages
import 'prismjs/components/prism-markup-templating';
import 'prismjs/components/prism-php';
import 'prismjs/components/prism-java';
// Option 1: Load a default theme (included in the 'prismjs' package)
import 'prismjs/themes/prism-okaidia.css';
// Option 2: Import an extra theme (included in the 'prism-themes' package)
import 'prism-themes/themes/prism-coldark-dark.css';
// Option 3: Load your own theme:
import 'path/to/your/theme/your-theme-name.css';
<pre><code class="language-javascript">const test = () => console.log('test!');</code></pre>